Videos
Videos are the core resource in VEO. All of the analysis, tag sessions, comments and AI insights revolve around the video resource. Here we'll look at how to create a video programatically.
Handled file types
VEO handles multiple types of video and audio. When uploading audio, the file is actually transcoded into video and may be visualised with a synthesized audio wav form video track depending on the transcoders current capacity. If the transcoders are busy, the video will instead include a black video track.
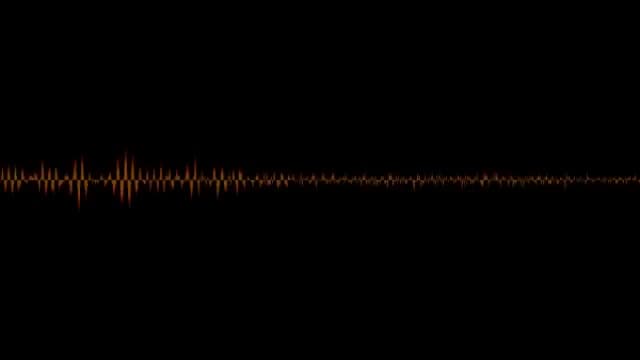
This is how a wave form looks when produced.
This will only be produced on audio file formats if the transcoders have capacity. If not they will convert the audio to video but posess a black video frame.
The video formats we handle are:
Mime Type | File Extension |
---|---|
VIDEO/MP4 | .mp4 |
VIDEO/QUICKTIME | .mov |
VIDEO/X-MS-WMV | .wmv |
VIDEO/X-MSVIDEO | .avi |
VIDEO/AVI | .avi |
VIDEO/MPEG | .mpeg |
VIDEO/X-MS-ASF | .asf |
VIDEO/VND.DLNA.MPEG-TTS | .mpeg |
AUDIO/MP3 | .mp3 |
AUDIO/MPEG | .mp3 |
AUDIO/WAV | .wav |
AUDIO/WAVE | .wav |
AUDIO/M4A | .m4a |
AUDIO/X-M4A | .m4a |
Creating videos
Create videos programatically is a multi step process. Our videos are hosted in Azure in isolated containers for each of our customers in a geolocation suited to your organisation's security strategy. It's for this reason that videos must be uploaded to us in three steps:
- Create a video asset by sending a
POST
request to the/videos
endpoint giving you a new video resourceid
- Aquire an upload token (a unique one-time use URL) by sending a
GET
request tovideos/{VideoId}/uploadtoken?mimetype=video/mp4
- Finally, upload the video to the upload token URL directly by sending a
PUT
request to the upload token URL returned in step 2.
Let's have a look at each of these steps in more detail.
Create a video
This endpoint creates a new video resource and sets it's state to 1 - Awaiting video token request
. More on the video statuses later.
The body of the post should be a JSON object with the following properties:
JSON Attributes
- Name
Title
- Type
- string
- Description
The title of the video as it will appear in VEO
- Name
RecordedStamp
- Type
- datetime
- Description
The time the video was recorded. If unsure just set this to the current date and time.
Response
The response should be a 200
status code, the body of which should be the video id
which you will need for the next step.
Request
curl --location 'https://api.veo.co.uk/api/videos/' \
--header 'Authorization: Bearer {token}' \
--header 'Content-Type: application/json' \
--data '{
"Title":"Principal Implementation Liaison",
"RecordedStamp":"2023-02-03T11:58:28.359Z"
}'
Get an upload token
Once you've created a video asset, you'll need to upload the video to us. This is done in two steps, the first of which is to get an upload token which is discussed here.
You get an upload token by making a GET request specifying the video ID in the URL and the mimetype of the original video in the query string.
Response
The response for this call will be a 200
and include a unique URL in the body to which you should upload your video. Be careful with the response as the URL is returned in the body wrapped in double quotes.
Request
curl --location 'https://api.veo.co.uk/api/videos/{VideoId}/uploadtoken?mimetype=video%2Fmp4' \
--header 'Authorization: Bearer {bearer}'
Upload your video to Azure
Now that you've got an upload token, you need to upload your video to Azure. To do this you need to send a PUT
request to the URL returned in the previous step.
Headers
This request isn't to the VEO API and so you don't need to include your Authorization bearer token as you do with other requests. You do however need to include a couple of extra headers specific to Azure.
- Name
x-ms-blob-type
- Type
- string
- Description
The value should be explictly set to
BlockBlob
- Name
Content-Type
- Type
- string
- Description
This should be the content type of the video (or audio) file.
Response
The response for this call will be a 201 - Created
with an empty body. At this point there's nothing further to do. The video will be picked up by our transcoders and formatted into multiple formats suitable for the web.
Request
curl --location --request PUT 'https://{{azurecontainer}}.blob.core.windows.net/videos/{{organisationId}}/{{userId}}/{{videoId}}/original.mp4?{{tokenSpecificQueryString}}' \
--header 'x-ms-blob-type: BlockBlob' \
--header 'Content-Type: video/mp4' \
--data '@/c:/Path/To/Video.mp4'
Transcoding
Once we receive your video it's queued for transcoding and picked up by one of the transcoders. Here the transcoder formats the video into a lower-resolution mp4 file suitable for streaming in the portal. We maintain a copy of the original for future AI analysis also but beyond this point only serve the transcoded versions back.
Throughout it's lifetime, a video can be in one of the following states:
- Awaiting video token request - you've created the video but not yet requested an upload token.
- Awaiting upload - you've requested an upload token but not yet uploaded the video.
- Awaiting transcode - The video has been received but not yet transcoded.
- Transcoding - A transcoder is actively working on transcoding the video.
- Transcoded - The video is ready to view in our platform.
- Error - An error occurred and the video will remain unavailable.
Watching/downloading videos
Similar to uploading a video, in order to watch or download a video, you need to obtain a download token for the video.
You do this by making a request GET
request then selecting from the aspect ratios returned to you with a token for each.
You can only obtain a download token after the video has been transcoded.
VideoPlayback Model
The model which is returned is as follows:
- Name
Id
- Type
- long
- Description
The ID of the video
- Name
Duration
- Type
- long
- Description
The duration of the video in seconds
- Name
Tokens
- Type
- Token[]
- Description
The different videos
Token model
- Name
Container
- Type
- string
- Description
Video type - usually mp4
- Name
Width
- Type
- int
- Description
The width of the video's aspect ratio
- Name
Height
- Type
- int
- Description
The height of the video's aspect ratio
- Name
TranscodePreset
- Type
- string
- Description
A name for the transcode preset which specifies the output resolution and type.
- Name
Url
- Type
- string
- Description
The one time use download url.
Request
curl --location 'https://api.veo.co.uk/api/videos/{VideoId}/downloadtoken' \
--header 'Authorization: Bearer {accesstoken}' \
Get all videos
Retrieving a list of all the videos you can see is done through the latest version of the get-all endpoint which returns a paginated list of videos.
Query string parameters
- Name
createdByMe
- Type
- bool
- Description
When set to true, this will return videos that you have created. If false, this will only return videos which have been shared with you or a group you belong to.
- Name
pageSize
- Type
- bool
- Description
- Name
pageNumber
- Type
- bool
- Description
- Name
orderByDirection
- Type
- bool
- Description
See pagination - orders based on the uploaded stamp.
Request
curl --location 'https://api.veo.co.uk/api/videos/v3/get-all?createdByMe=true&pageSize=20&pageNumber=1&orderByDirection=DESC&orderBy=UPLOADEDSTAMP' \
--header 'Authorization: Bearer {accesstoken}' \
Other endpoints
For other endpoints please visit the swagger documentation.